ML Beginner's Guide To Build Pneumonia Detection Model
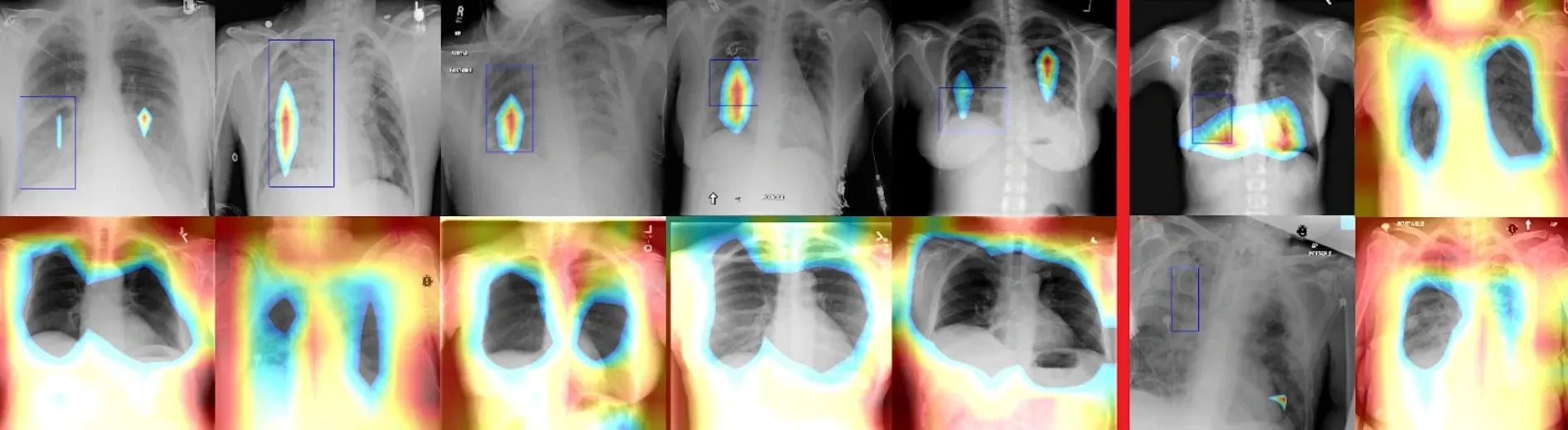
Introduction
Pneumonia is a severe respiratory infection affecting the lungs, often caused by bacteria, viruses, or fungi. Early detection plays a crucial role in effective treatment and patient care. In this tutorial, we'll explore how to build a pneumonia detection system using Machine Learning and Image Processing techniques. We will use a dataset containing chest X-ray images of patients with and without pneumonia. We'll create a machine learning model using Support Vector Machines (SVM) to classify these images and detect pneumonia. The dataset contains three main subsets: training, validation, and testing data.
About Dataset
The dataset used for pneumonia detection consists of chest X-ray images collected from pediatric patients aged one to five years old at the Guangzhou Women and Children’s Medical Center in Guangzhou, China. The dataset is organized into three main folders: train, test, and validation, with subfolders for each image category: Pneumonia and Normal. It comprises a total of 5,863 JPEG images and includes two distinct categories: Pneumonia and Normal.
Context and Image Details
Image Categories: The images are classified into two categories: Pneumonia and Normal.
Pneumonia Types: The Pneumonia category includes subtypes—Bacterial and Viral pneumonia. Bacterial pneumonia typically exhibits focal lobar consolidation, whereas viral pneumonia manifests with a more diffuse 'interstitial' pattern in the lungs.
Quality Control: Images underwent initial screening for quality control to remove any low-quality or unreadable scans, ensuring the dataset's integrity and reliability for analysis.
Diagnostic Grading: Expert physicians evaluated and graded the diagnoses of the chest X-ray images before they were used to train the AI system. Additionally, a third expert checked the evaluation set to account for any potential grading errors, ensuring accuracy and reliability in the dataset's annotations.
Dataset Composition
Image Quantity: The dataset comprises a significant number of chest X-ray images (5,863 in total), which is essential for training robust machine learning models.
Data Split: It is divided into three subsets—training, testing, and validation—ensuring separate sets for model training, evaluation, and validation purposes.
Age Group: The dataset focuses on pediatric patients aged one to five years old, highlighting the relevance of early diagnosis and management of pneumonia in young children.
Clinical Context: The chest X-ray images were obtained as part of routine clinical care for these pediatric patients, emphasizing the real-world applicability of the dataset.
Hands-on Tutorial
1. Importing Libraries
Libraries like NumPy, scikit-learn (with SVM, metrics, GridSearchCV), TensorFlow (for ImageDataGenerator), PIL (for image handling), and Matplotlib (for visualization) are essential. These libraries provide functionalities for data handling, machine learning, image processing, and result visualization.
import numpy as np
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score, precision_score, recall_score, roc_auc_score
from sklearn.model_selection import GridSearchCV
from sklearn.pipeline import make_pipeline
from sklearn.preprocessing import StandardScaler
from tensorflow.keras.preprocessing.image import ImageDataGenerator
import PIL
import matplotlib.pyplot as plt
2. Loading and Preprocessing Images
Image Loading: Here, an X-ray image of a normal chest is loaded using the PIL library. In real-world healthcare scenarios, large databases of medical images are used for training and validation purposes.
Data Augmentation: The training generator from ImageDataGenerator applies transformations like rotation, zoom, and flips to generate more diverse training images. This augmentation technique helps in creating a robust model by exposing it to various scenarios that might be present in real medical images.
# Load images
image_normal = PIL.Image.open("/kaggle/input/chest-xray-
pneumonia/chest_xray/train/NORMAL/IM-0115-0001.jpeg")
# Data generators with augmentation
training_generator = ImageDataGenerator(
rescale=1/255,
rotation_range=15,
zoom_range=0.2,
horizontal_flip=True,
vertical_flip=True
)
validation_generator = ImageDataGenerator(rescale=1/255)
test_generator = ImageDataGenerator(rescale=1/255)
# Load the training data for the neural network
training_dir = "/kaggle/input/chest-xray-pneumonia/chest_xray/train/"
data_train = training_generator.flow_from_directory(
training_dir,
target_size=(120, 120),
batch_size=8,
class_mode="binary"
)
# Load the validation and test data for the neural network
valid_dir = "/kaggle/input/chest-xray-pneumonia/chest_xray/val/"
data_valid = validation_generator.flow_from_directory(
valid_dir,
target_size=(120, 120),
batch_size=8,
class_mode="binary"
)
test_dir = "/kaggle/input/chest-xray-pneumonia/chest_xray/test/"
data_test = test_generator.flow_from_directory(
test_dir,
target_size=(120, 120),
batch_size=8,
class_mode="binary"
)
3. Preparing Data for SVM
Flattening Image Data: The image data is flattened to be compatible with SVM. For each image, the pixels are flattened into a 1D array. This process converts the image matrix into a feature vector, making it suitable for SVM, which requires 1D input.
# Flatten the image data for SVM
X_train_svm = np.array([data_train[i][0][0].flatten() for i in range(len(data_train))])
y_train_svm = np.array([data_train[i][1][0] for i in range(len(data_train))])
X_valid_svm = np.array([data_valid[i][0][0].flatten() for i in range(len(data_valid))])
y_valid_svm = np.array([data_valid[i][1][0] for i in range(len(data_valid))])
X_test_svm = np.array([data_test[i][0][0].flatten() for i in range(len(data_test))])
y_test_svm = np.array([data_test[i][1][0] for i in range(len(data_test))])
4. Creating and Training SVM Model
SVM Model Creation: An SVM model is created using make_pipeline()
, combining a StandardScaler (for feature scaling) and SVC (Support Vector Classifier) with probability=True to enable probability estimates.
Hyperparameter Tuning: Hyperparameters such as C (regularization parameter), kernel type, gamma, and class weights are explored using GridSearchCV. This method performs cross-validation to find the best combination of hyperparameters.
# Create an SVM model
svm_model = make_pipeline(StandardScaler(), SVC(probability=True))
# Define an extended hyperparameter grid
param_grid = {
'svc__C': [0.001, 0.01, 0.1, 1, 10, 100],
'svc__kernel': ['linear', 'poly', 'rbf', 'sigmoid'],
'svc__gamma': ['scale', 'auto'],
'svc__class_weight': [None, 'balanced']
}
# Perform GridSearchCV to find the best hyperparameters
grid_search_svm = GridSearchCV(svm_model, param_grid, cv=5, scoring='accuracy', n_jobs=-1)
grid_search_svm.fit(X_train_svm, y_train_svm)
# Get the best model
best_svm_model = grid_search_svm.best_estimator_
5. Evaluating the Model
Model Evaluation: The model is evaluated on the test set using various metrics like accuracy, precision, recall, and AUC (Area Under the ROC Curve).
# Evaluate the SVM model on the test set
y_test_pred_svm = best_svm_model.predict(X_test_svm)
test_accuracy_svm = accuracy_score(y_test_svm, y_test_pred_svm)
test_precision_svm = precision_score(y_test_svm, y_test_pred_svm)
test_recall_svm = recall_score(y_test_svm, y_test_pred_svm)
test_auc_svm = roc_auc_score(y_test_svm, best_svm_model.predict_proba(X_test_svm)[:, 1])
print(f"SVM Test Accuracy: {test_accuracy_svm}")
print(f"SVM Test Precision: {test_precision_svm}")
print(f"SVM Test Recall: {test_recall_svm}")
print(f"SVM Test AUC: {test_auc_svm}")
6. Visualizing Predictions
The model's predictions on a batch from the test set are displayed alongside the respective chest X-ray images.
# Display predictions on a batch from the test set
x, y = data_test.next()
predictions_svm = best_svm_model.predict_proba(X_test_svm)
# Display results
for j in range(8):
plt.imshow(x[j])
plt.show()
print("Probability of pneumonia in this image (SVM): ", predictions_svm[j, 1])
Result
Importance of Pneumonia Detection in Medical and Healthcare Industry
1. Early Diagnosis and Triage
Timely Intervention: Early identification of pneumonia allows healthcare providers to initiate appropriate treatments promptly, potentially preventing complications and reducing the severity of the illness.
Optimized Resource Allocation: By diagnosing pneumonia early, medical resources such as hospital beds, medications, and intensive care units (ICUs) can be utilized more efficiently, optimizing patient care.
Prevention of Disease Spread: Swift diagnosis helps in implementing isolation measures, reducing the risk of transmission within healthcare facilities and the community.
2. Remote Healthcare
Access to Specialized Care: In remote or underserved areas lacking immediate access to healthcare professionals, AI-driven systems can act as a preliminary diagnostic tool, providing initial assessments and guidance to individuals in need.
Telemedicine Support: Through telemedicine platforms, AI-enabled tools can connect patients in remote locations with specialists, allowing remote monitoring and consultation, leading to better healthcare outcomes.
Reduced Travel and Costs: AI-based diagnostic tools reduce the need for patients to travel long distances for basic healthcare needs, saving both time and expenses.
3. Screening Programs
Population Health Management: Automated screening processes assist in identifying individuals at risk for pneumonia and other respiratory conditions. Targeted interventions, such as vaccination campaigns or early interventions, can be implemented for high-risk groups.
Public Health Surveillance: AI-driven screening programs contribute to public health surveillance by continuously monitoring and identifying patterns of pneumonia outbreaks or epidemics, aiding in disease control and prevention strategies.
Resource Allocation for Prevention: Identifying at-risk individuals enables healthcare organizations and policymakers to allocate resources efficiently for preventive measures, such as health education programs and community interventions.
Frequently Asked Questions
1.Can machine learning improve pneumonia image detection?
Machine learning, especially deep learning models like convolutional neural networks (CNNs), vastly improves pneumonia image detection by learning complex patterns within chest X-ray images. Trained on extensive datasets, these models accurately discern abnormalities indicating pneumonia, enabling rapid and reliable automated analysis. This technology enhances diagnostic precision, expedites patient care, and holds promise in improving healthcare outcomes by aiding early and accurate pneumonia detection.
2. Can deep learning detect pneumonia using X-rays?
Yes, deep learning, particularly convolutional neural networks (CNNs), has demonstrated remarkable success in detecting pneumonia using X-ray images. CNNs can analyze chest X-ray images to identify patterns and abnormalities associated with pneumonia, distinguishing between normal and pneumonia-affected lungs with high accuracy. This technology has shown promise in automating and enhancing the diagnostic process, aiding healthcare professionals in the efficient and accurate detection of pneumonia from X-ray images.
3. Can computer-aided techniques be used to detect pneumonia?
Absolutely, computer-aided techniques, especially those employing machine learning and deep learning algorithms, are widely used in detecting pneumonia. These techniques analyze medical images such as X-rays using sophisticated algorithms that can identify patterns and abnormalities indicative of pneumonia. By leveraging AI-driven models, computer-aided techniques assist radiologists and clinicians in interpreting images more accurately and efficiently, thereby improving diagnostic accuracy and enabling early detection of pneumonia, ultimately leading to more effective patient care.
Book our demo with one of our product specialist
Book a Demo